JavaScript Framework Overload
In 2010, Google released AngularJS. Within a couple years, it was the front-end web framework to use when building a “Single Page App” experience. Angular’s Model-View-* approach was familiar enough to web developers and added magical data binding to speed up development of rich, responsive interfaces.
After AngularJS reached its peak dominance, it felt like a new JavaScript framework popped up once a month. One of these was React, rethinking the way UI is developed and rendered. It was created in 2013 but because of the oversaturation of JavaScript frameworks around that time, React didn’t gain widespread popularity until the end of 2015. This graph really helps visualize the trends in the context of technologies mentioned in job postings.
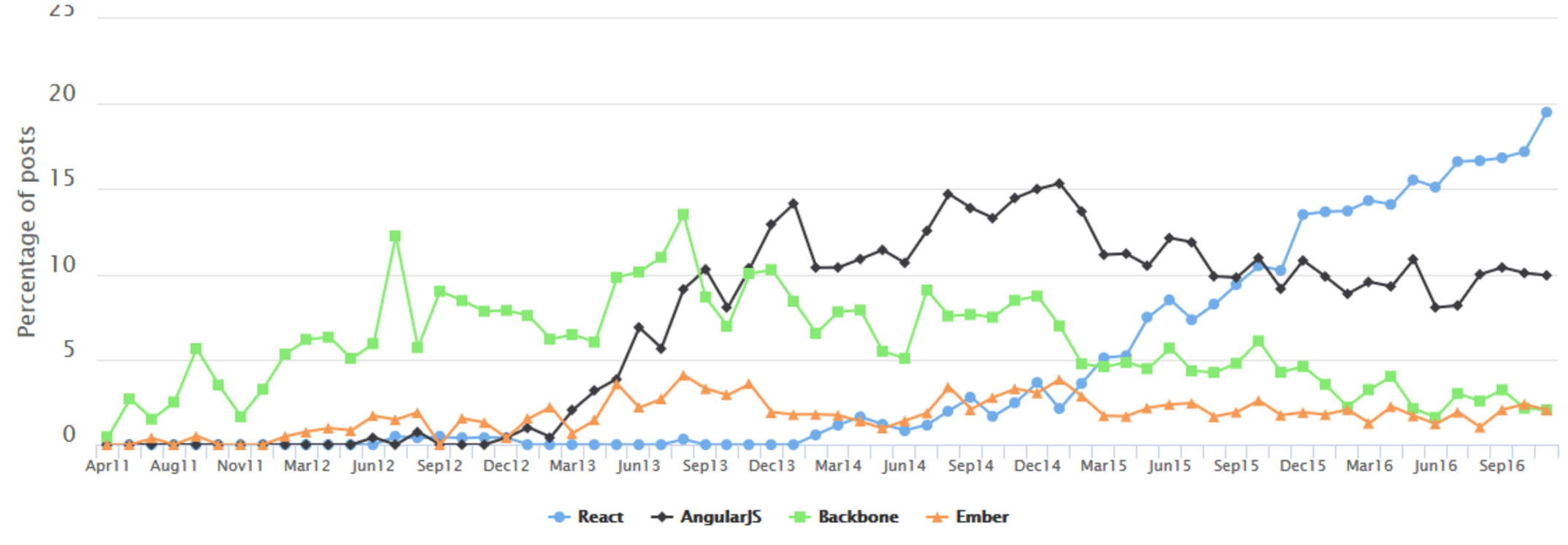
What makes React unique?
React embodied the concept that UI can be distilled down to components as a function of state. Every piece of a web application can be split into smaller maintainable components, which take in some type of state (data) as input, and render UI (HTML) as output. This simple idea—which gained traction in front-end development with help from React—changed front-end web application development and is a common practice today.
React was the first to implement a “virtual DOM,” a diffing mechanism to store a copy of the real DOM, analyze changes in the application’s state, and only change the necessary parts before re-rendering HTML. This performance enhancement quickly caught on and is now also used in Ember and Vue.
UI as Components
In many ways, web/UI developers are already used to the idea of components—we use things like <button type="submit">
and <input type="text">
all the time. React components are meant to divide parts of an app or website into small, maintainable pieces that are easy to reason about, and can be reused wherever possible.
As an example, let’s create a <PlayingCard>
component (simplified with two numbers on opposite corners and a suit graphic in the center):
// PlayingCard.js (component)
const PlayingCard = ({suit, number}) => (
<div className="playing-card">
<span className="playing-card__number">{number}</span>
<div className="playing-card__img">
<img src={`img/suits/${suit}.png`} alt={suit} />
</div>
<span className="playing-card__number—inverted">{number}</span>
</div>
);
// data from somewhere
const cards = [
{
suit: 'diamond',
number: 'J'
},
{
suit: 'spade',
number: 'Q'
},
{
suit: 'heart',
number: '5'
},
{
suit: 'club',
number: '2'
}
];
// render to DOM
{cards.map(card => (
<PlayingCard suit={card.suit} number={card.number} />
))}
The React Ecosystem
React has always been a smaller library focused primarily on the UI. One of its main selling points is its (relatively) small size and abstraction from how the rest of the application is structured. It can be dropped into multiple contexts (even within apps built with other JS frameworks), and developers can manage their data however they prefer.
When trying to build new web applications with React, this led to confusion around tools for quite some time, and there were more questions than answers. What about routing? Data modeling and application state management? Whose configuration (and how much) do I need to just get going? With React’s flexibility comes no “right way” to do things—until certain projects and ideas began bubbling to the surface.
Over the past couple years, Facebook and open source communities have addressed a lot of these issues, and today React tooling is fairly well agreed-upon. The following tools are great starting places to get going with React apps, while none are binding or mandatory:
Routing
Ryan Florence and the folks at React Training maintain React Router and keep it right in step with React updates. It treats routes as React components (noticing a theme?), which feels familiar to developers already using React.
State management
When keeping track of state across multiple React components/routes, Redux has become the state manager of choice, providing a simple way to store state for access anywhere. Redux was written by Dan Abramov, who was later hired by Facebook and is an active maintainer of React and its surrounding tools.
Bootstrapping
In response to tools like Yeoman and the Ember CLI, Facebook built Create React App to reduce configuration and setup for React development. Developers can get started by running $ create-react-app myproject
, and a simple project will be bootstrapped with a development server, transpiling, and dev/test/build scripts.
Getting Started
There are a lot of fantastic resources around the web for any developer who wants to get started in React. To really grasp React concepts, I encourage using it on small side projects and trying out the tools listed above. The following video tutorials are incredibly helpful for guided explanation and practice:
- Frontend Masters: Complete Intro to React (Brian Holt)
- React Training: React Fundamentals (Tyler McGinnis)
- React for Beginners (Wes Bos)
- Egghead: Getting Started with Redux (Dan Abramov)
This is a lot of information to absorb and should give you a great starting point to learn React as you start building small-scale projects.
Questions? Reach me on Twitter: @markpalfreeman
As JavaScript developers grow in their field, adding a popular framework like React to their skill set is one of the best things they can do.
To continue to train in the most relevant skills for the tech industry, we’re excited to teach React in Code 401: Advanced Software Development in Full-Stack JavaScript!
Ready to add React to your toolkit? Apply »